# Monty Hall Problem Simulation ## Demo You can view the live demo of the simulation [here](https://monty-hall-simulation.vercel.app/). 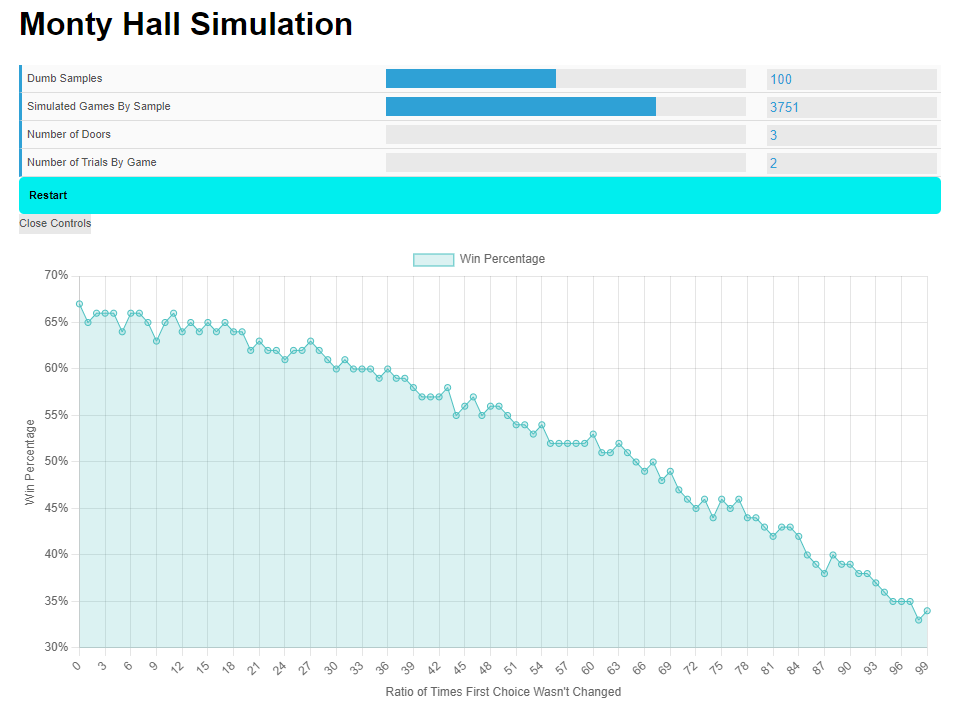 ## Overview This project is a vanilla JavaScript simulation of the Monty Hall problem, a famous probability puzzle based on a game show scenario. The simulation demonstrates the counterintuitive result that switching choices in the game increases the chances of winning. ## The Monty Hall Problem In the Monty Hall problem, a contestant is faced with three doors. Behind one door is a car (the prize), and behind the other two doors are goats. After the contestant chooses a door, the host, Monty Hall, reveals a goat behind one of the other two doors. The contestant is then given a choice: stick with their original door or switch to the remaining unopened door. The correct strategy is to switch doors, which gives a 2/3 chance of winning the car, compared to a 1/3 chance if they stick with their initial choice. ## Features - **Interactive GUI**: Adjust the number of doors, games, trials, and samples using the DAT.GUI interface. - **Real-time Simulation**: The simulation runs in real-time, updating the results dynamically. - **Graphical Display**: The results are plotted using Chart.js, displaying the win percentage based on the ratio of times the first choice wasn't changed. ## Project Structure - **`three-door-problem.js`**: Contains the core logic for the Monty Hall simulation, including the functions to simulate the game and calculate the win percentages. - **`index.html`**: The HTML file that sets up the GUI and chart display. - **`style.css`**: Basic styling for the project. - **`main.js`**: The main JavaScript file that integrates the simulation logic with the GUI and Chart.js. ## Usage 1. Clone the repository: bash Copia el codi `git clone https://github.com/rfranr/monty-hall-simulation.git` 2. Open `index.html` in your browser. 3. Use the GUI on the left side of the screen to adjust the parameters: - **Samples**: Number of samples to simulate. - **Simulated Games By Sample**: Number of games per sample. - **Number of Doors**: Number of doors in the game (default is 3). - **Number of Trials By Game**: Number of trials (choices) allowed per game. 4. The chart on the right side will update in real-time, showing the win percentage based on the cognitive dissonance ratio (the ratio of times the first choice wasn't changed). ## Code Explanation - **Simulation Setup**: The `getChallenge()` function creates the doors with one prize and the rest as goats. The `choseDoor()` function allows the contestant to pick a door randomly. - **Stats Calculation**: The `stats()` function runs multiple simulations, adjusting the cognitive dissonance ratio (how often the contestant sticks to their first choice) and records the win percentage for each sample. - **GUI and Chart Integration**: The GUI is set up using DAT.GUI, allowing users to change the parameters. The results are displayed using Chart.js, showing the relationship between sticking to the first choice and the win percentage.